REST API Integration Guide for Beginners
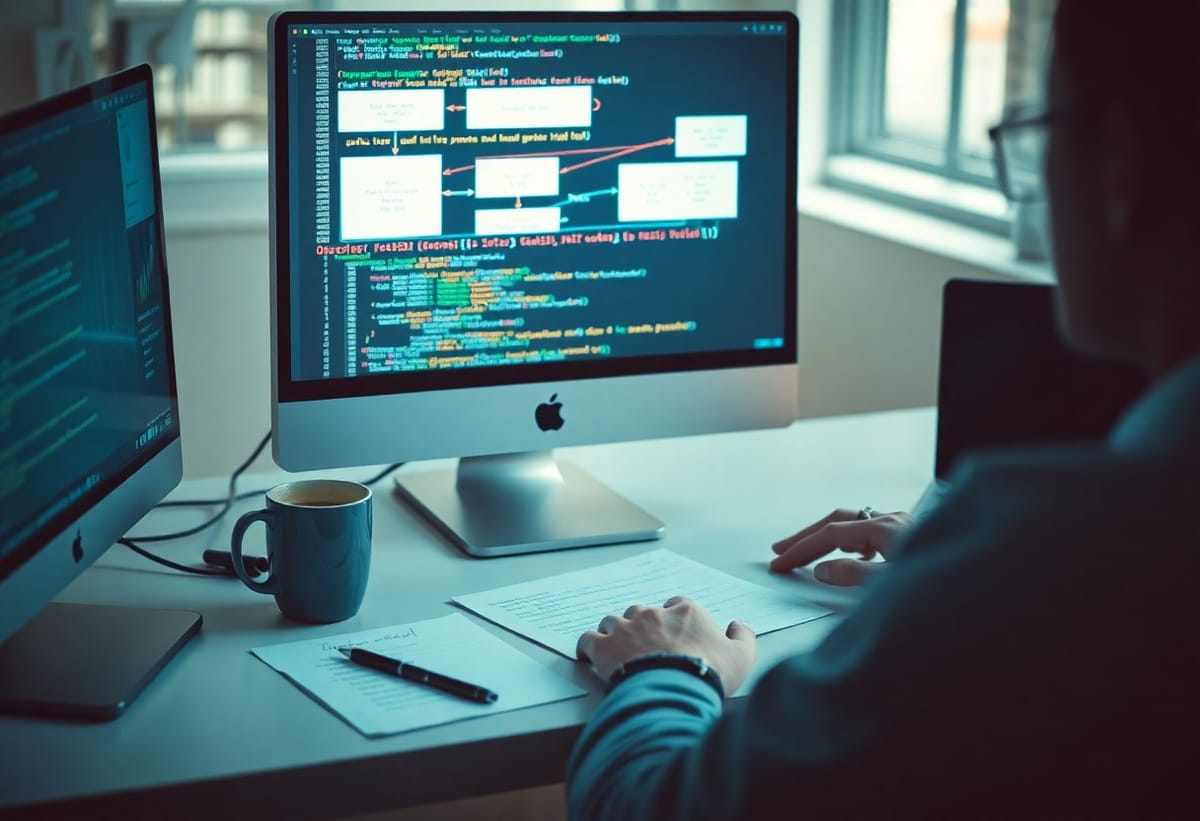
Integration with REST APIs can transform your applications from isolated systems into powerful, interconnected platforms. Whether you're building a mobile app, web service, or enterprise solution, understanding how to work with REST APIs will expand your development capabilities significantly. In this guide, you'll learn the fundamental concepts of REST API integration, explore practical examples, and discover best practices that will help you confidently implement API connections in your projects.
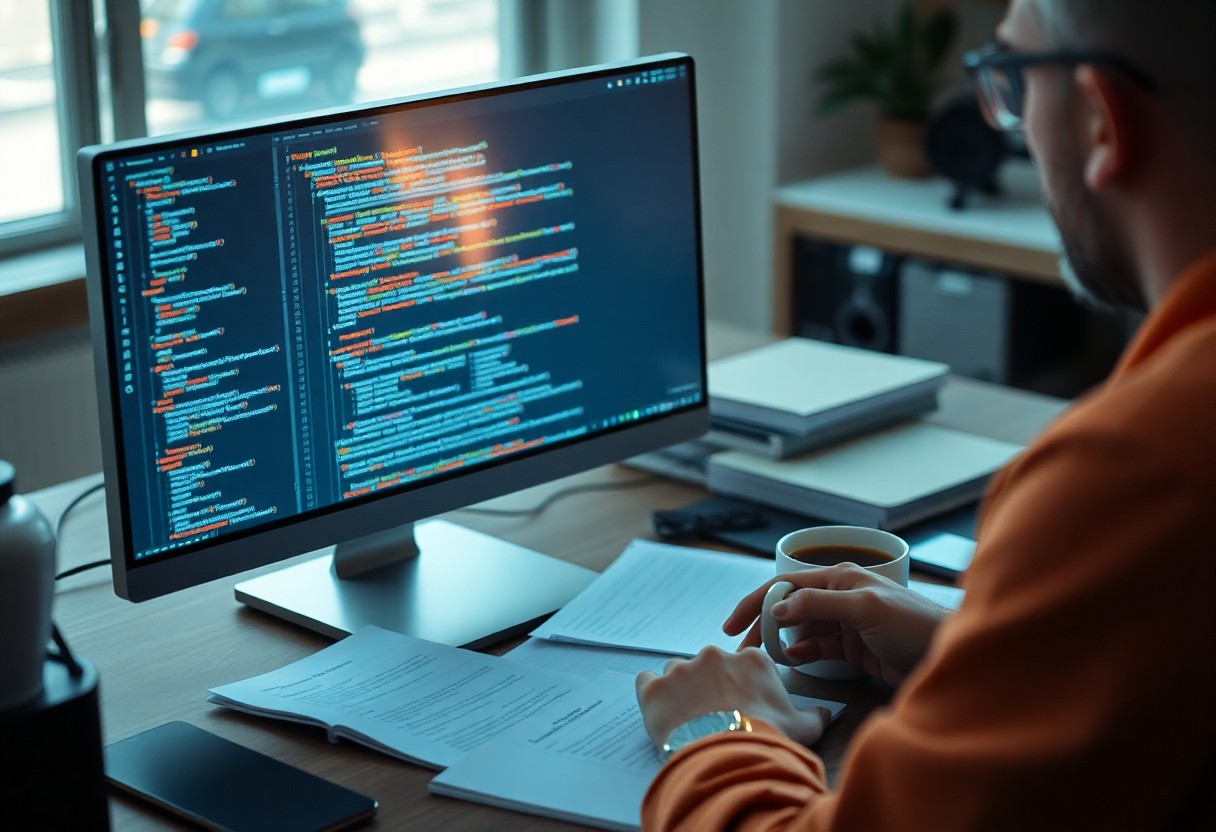
Key Takeaways:
- REST APIs use standard HTTP methods (GET, POST, PUT, DELETE) to communicate between client and server, making them straightforward to implement and understand
- Authentication and security measures like API keys, tokens, or OAuth are vital components for protecting your API endpoints and managing access
- Proper error handling and status codes help developers quickly identify and resolve issues while maintaining clear communication between systems
- JSON is the most common data format for REST APIs, offering a lightweight and readable structure for data exchange
- Testing your API endpoints with tools like Postman or cURL ensures reliability and helps document expected behaviors for other developers
Understanding REST APIs
Your journey into REST APIs begins with grasping their fundamental role in modern web development. These architectural principles enable seamless communication between different software systems, allowing you to build robust and scalable applications that can interact with various services and databases.
What is a REST API?
While developing web applications, you'll encounter REST (Representational State Transfer) APIs as standardized interfaces that allow different systems to communicate over HTTP. These APIs use simple HTTP methods like GET, POST, PUT, and DELETE to perform operations on resources, making them easy to implement and maintain.
Types of REST APIs
There's a variety of REST APIs you can work with, each serving different purposes in your applications:
- Public APIs: Available for general use
- Private APIs: Internal system communication
- Partner APIs: Limited external access
- Composite APIs: Combining multiple services
- Recognizing these types helps you choose the right API for your needs
API Type | Use Case |
---|---|
Public | Social media integration |
Private | Internal microservices |
Partner | B2B solutions |
Composite | Complex workflows |
Custom | Specific business needs |
Understanding the different API types allows you to design better integrations and choose the most suitable approach for your specific use case. Each type offers unique advantages and security considerations that you'll need to factor into your development process.
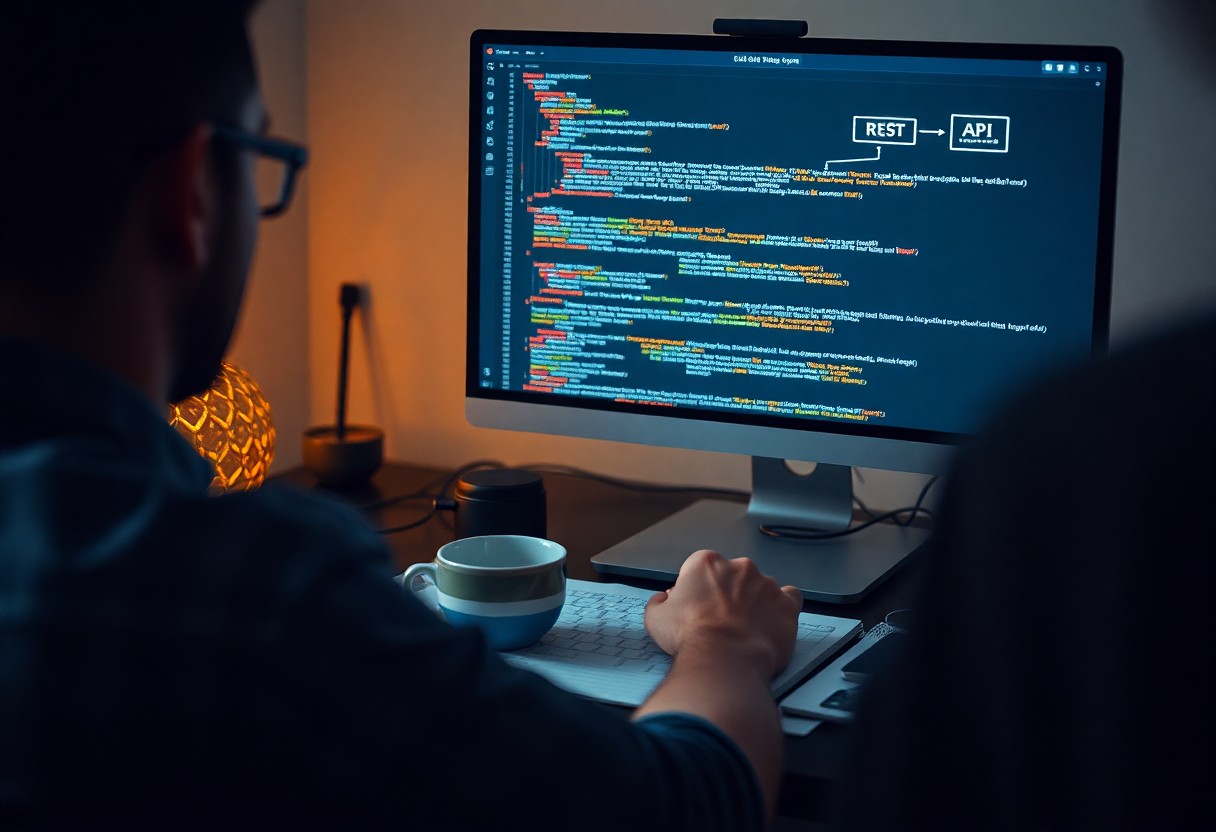
Step-by-Step Guide to REST API Integration
Any successful REST API integration follows a structured approach that ensures smooth implementation and reliable results. Here's your comprehensive roadmap to getting started with API integration:
Environment Setup | Configure development tools and dependencies |
Authentication | Obtain and manage API keys/tokens |
Request Testing | Validate API endpoints and responses |
Error Handling | Implement robust error management |
Setting Up Your Environment
With the right tools in place, you'll streamline your API integration process. Start by installing a reliable REST client like Postman or Insomnia, set up your preferred programming environment, and ensure you have the necessary libraries for making HTTP requests in your chosen language.
Making Your First API Call
With your environment ready, you can begin making API calls. Select a simple endpoint from your chosen API, prepare your request headers, and send a GET request to fetch data. Monitor the response status and examine the returned JSON or XML data structure.
Plus, you'll want to explore different request methods (GET, POST, PUT, DELETE) and understand how to handle query parameters, request bodies, and response parsing. Test your API calls with various scenarios to ensure robust implementation and proper error handling in your code.
Key Factors to Consider
To ensure successful API integration, you need to focus on several important elements. Understanding authentication methods, rate limits, data formats, and error handling will help you build robust connections. Your implementation should account for versioning, documentation quality, and security protocols. Perceiving these factors early in development prevents common integration pitfalls.
Authentication Methods
For securing your API requests, you'll encounter various authentication mechanisms. API keys, OAuth tokens, and JWT (JSON Web Tokens) represent the most common approaches. Your choice depends on your security requirements and the API provider's specifications. Basic authentication might work for simple projects, while OAuth 2.0 offers more sophisticated security features for complex applications.
Rate Limits and Usage Quotas
Usage restrictions control how frequently you can make API calls within specific timeframes. Your application needs to respect these limits to maintain uninterrupted service. Most APIs implement tiered access levels, where higher tiers allow more requests per second or day.
Factors affecting your rate limit management include request throttling, concurrent connections, and handling rate limit responses. You should implement proper error handling and request queuing to stay within allocated quotas. Your application can benefit from caching frequently requested data and implementing exponential backoff strategies when approaching limits.
Tips for Successful REST API Integration
Now that you've grasped the basics of REST APIs, it's time to focus on implementing them effectively in your projects. Follow these necessary guidelines to ensure smooth integration:
- Always read the API documentation thoroughly
- Test your endpoints in a development environment
- Implement proper error handling
- Use appropriate authentication methods
- Monitor API rate limits
Knowing these fundamentals will help you build robust and reliable API integrations.
Best Practices
If you want to maintain clean and efficient API integrations, adopt a systematic approach. Keep your API keys secure and never expose them in your code. Cache responses when possible to reduce unnecessary calls. Use version control for your API implementations, and maintain consistent error handling across your application. Document any custom implementations or workarounds you develop.
Common Pitfalls to Avoid
One of the most frequent mistakes you can make is assuming all APIs follow the same standards. Different providers implement REST principles differently, and overlooking these variations can lead to integration issues. Pay attention to response formats, authentication requirements, and rate limiting policies specific to each API.
To prevent problems in your API integrations, you need to validate all incoming data, handle timeouts appropriately, and implement retry mechanisms for failed requests. Make sure you're using the correct content types and HTTP methods for each endpoint. Keep your error handling comprehensive yet specific to provide meaningful feedback when issues occur.
Pros and Cons of Using REST APIs
Once again, understanding the advantages and limitations of REST APIs will help you make informed decisions about their implementation in your projects. Here's a comprehensive breakdown of the key benefits and potential drawbacks:
| Stateless architecture | Limited built-in securityPlatform independence | Can be over-fetching dataLanguage agnostic | No real-time supportScalability | Multiple endpoint managementCaching capability | Version control challengesEasy to understand | Network latency impactWide adoption | Documentation requirementsFlexible data formats | Limited batch operations
Advantages of REST APIs
Using REST APIs provides you with remarkable flexibility in developing modern applications. You can easily integrate different services, scale your applications horizontally, and leverage the stateless nature of REST to build robust systems that handle high traffic efficiently.
Disadvantages of REST APIs
If you're working with complex applications, REST APIs might present certain challenges. You'll need to handle multiple endpoints, manage versioning, and deal with potential over-fetching of data, which can impact your application's performance.
Another significant consideration is the security aspect of REST APIs. You'll need to implement additional security measures, handle authentication properly, and ensure proper error handling across all endpoints. The lack of built-in state management means you'll have to handle session management separately in your application.
Summing up
Conclusively, your journey into REST API integration equips you with necessary skills for modern web development. By following this guide, you've learned how to make HTTP requests, handle responses, authenticate your applications, and manage endpoints effectively. Understanding these fundamentals allows you to connect and interact with various web services confidently. As you continue to build applications, you'll find these REST API concepts becoming second nature, enabling you to create more sophisticated and interconnected solutions that meet your development needs.
FAQ
Q: What is a REST API and why should I use it?
A: A REST API (Representational State Transfer) is a set of rules and conventions for building web services that allow different applications to communicate with each other. You should use REST APIs because they're widely adopted, easy to understand, and provide a standardized way to exchange data between client and server applications using HTTP methods like GET, POST, PUT, and DELETE.
Q: How do I authenticate my REST API requests?
A: Common authentication methods include API keys, OAuth tokens, and JWT (JSON Web Tokens). You typically include these credentials in your request headers. For example, you might add an 'Authorization' header with a bearer token or include an API key as a query parameter. Always follow the specific authentication requirements provided by the API documentation.
Q: What are the basic HTTP methods used in REST APIs?
A: The main HTTP methods are: GET (retrieve data), POST (create new data), PUT (update existing data), DELETE (remove data), and PATCH (partial update). Each method serves a specific purpose in CRUD (Create, Read, Update, Delete) operations. For example, use GET to fetch user information, POST to create a new user, PUT to update user details, and DELETE to remove a user.
Q: How do I handle errors in REST API responses?
A: REST APIs use HTTP status codes to indicate the result of requests. Common codes include 200 (success), 201 (created), 400 (bad request), 401 (unauthorized), 404 (not found), and 500 (server error). Always implement error handling in your code to catch and process these status codes appropriately. The response body usually contains detailed error messages and additional information about what went wrong.
Q: What tools can I use to test REST APIs?
A: Popular tools for testing REST APIs include Postman (a user-friendly GUI tool for sending requests), cURL (command-line tool), Swagger UI (interactive documentation and testing), and Insomnia (another GUI-based testing tool). These tools allow you to send requests, examine responses, set headers, and debug API interactions. Start with Postman if you're new to API testing, as it offers a gentle learning curve and comprehensive features.